You are learning Power Query in MS Excel
How to perform data validation and error handling in Power Query?
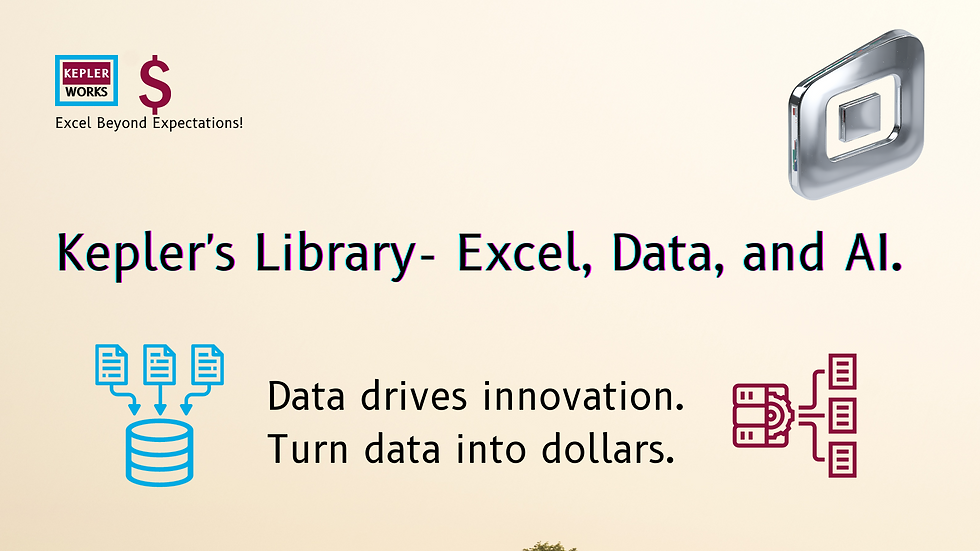
Performing data validation and error handling in Power Query involves several steps to ensure data integrity and manage errors effectively. Here's how you can do it:
Data Validation
1. Check for Null Values:
- Use the `Replace Values` feature to replace null values with a default value or remove them if necessary.
- Use the `Remove Rows` feature to remove rows with null values: `Home` tab > `Remove Rows` > `Remove Rows with Errors` or `Remove Blank Rows`.
2. Data Type Validation:
- Ensure each column has the correct data type.
- Select a column and go to the `Transform` tab > `Data Type` and choose the appropriate type (e.g., Text, Number, Date).
3. Conditional Column for Validation:
- Create a new conditional column that flags rows with invalid data.
- Go to the `Add Column` tab > `Conditional Column`.
- Define conditions that check for valid data (e.g., if a number column is within a certain range).
4. Custom Functions for Validation:
- Write custom functions to perform complex validation.
- Use the `Advanced Editor` to create custom M code functions that validate data based on your criteria.
Error Handling
1. Error Checking:
- Use the `Remove Errors` feature to eliminate rows with errors: `Home` tab > `Remove Rows` > `Remove Rows with Errors`.
- Alternatively, create a separate query that extracts rows with errors for review: `Add Column` > `Column From Examples` > `Extract Errors`.
2. Replace Errors:
- Replace errors with a default value using the `Replace Errors` feature.
- Select the column, then go to the `Transform` tab > `Replace Errors`.
3. Try and Otherwise Function:
- Use the `try` and `otherwise` functions in M code to handle errors gracefully.
- In the `Advanced Editor`, you can write code like:
```m
try [column] otherwise "Default Value"
```
4. Conditional Columns for Error Handling:
- Create a conditional column that identifies and flags errors.
- Go to `Add Column` > `Conditional Column` and set up conditions to check for errors.
Example of M Code for Validation and Error Handling
Here's an example of how you can use M code to handle errors and validate data:
```m
let
Source = Excel.Workbook(File.Contents("C:\path\to\file.xlsx"), null, true),
Data = Source{[Name="Sheet1"]}[Data],
// Replace null values with a default
ReplaceNulls = Table.ReplaceValue(Data, null, "Default Value", Replacer.ReplaceValue, {"Column1"}),
// Change data type and handle type errors
ChangeType = Table.TransformColumnTypes(ReplaceNulls, {{"Column1", type number}}),
// Handle type conversion errors
HandleErrors = Table.TransformColumns(ChangeType, {{"Column1", each try _ otherwise -1}}),
// Add a validation column
ValidationColumn = Table.AddColumn(HandleErrors, "Validation", each if [Column1] < 0 then "Error" else "Valid")
in
ValidationColumn
```
By implementing these techniques, you can ensure robust data validation and effective error handling in Power Query.